串处理语言翻译
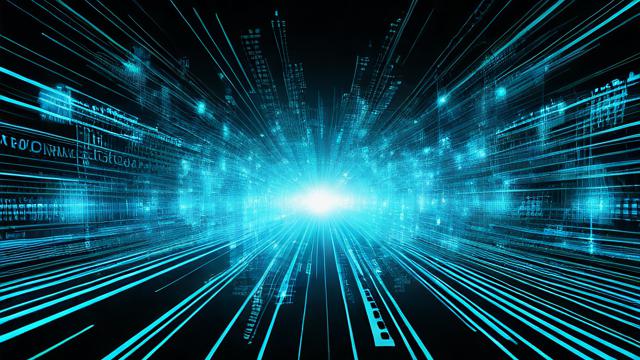
在计算机科学中,字符串处理是一种常见的操作,无论是在文本编辑、数据清洗还是自然语言处理等领域,对字符串的操作都是不可或缺的一部分,本文将详细介绍几种常见的字符串处理方法,包括查找、替换、分割和连接等,并通过实例代码展示如何在Python中实现这些操作。
字符串查找
1.1 使用find()
方法
find()
方法用于查找子字符串在父字符串中的位置,如果找到,则返回子字符串的起始索引;如果未找到,则返回-1。
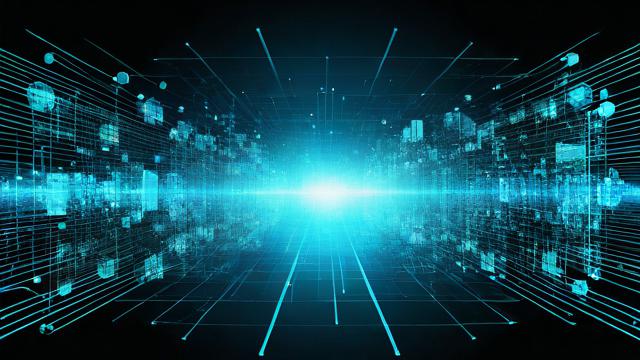
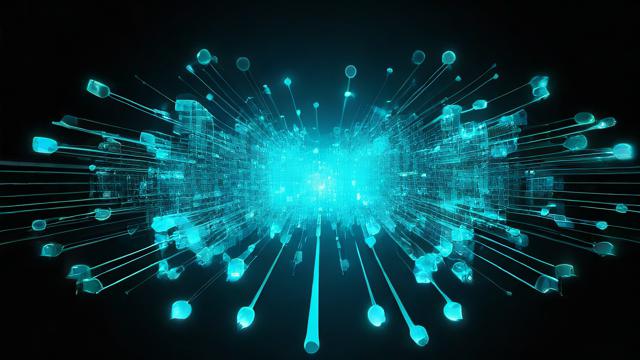
示例:
text = "Hello, world!" position = text.find("world") print(position) # 输出: 7
1.2 使用index()
方法
index()
方法与find()
类似,但如果找不到子字符串,则会引发ValueError异常。
示例:
text = "Hello, world!" try: position = text.index("Python") except ValueError: print("Substring not found") 输出: Substring not found
字符串替换
2.1 使用replace()
方法
replace()
方法用于将字符串中的某个子字符串替换为另一个子字符串,可以指定替换的次数,如果不指定,则替换所有匹配项。
示例:
text = "Hello, world! Hello, everyone!" new_text = text.replace("Hello", "Hi") print(new_text) # 输出: Hi, world! Hi, everyone!
字符串分割
3.1 使用split()
方法
split()
方法用于将字符串按照指定的分隔符进行分割,返回一个列表,如果不指定分隔符,则默认按空格分割。
示例:
text = "apple, banana, cherry" fruits = text.split(", ") print(fruits) # 输出: ['apple', 'banana', 'cherry']
字符串连接
4.1 使用+
运算符
直接使用+
运算符可以将两个或多个字符串连接起来。
示例:
part1 = "Hello" part2 = "world" full_text = part1 + ", " + part2 + "!" print(full_text) # 输出: Hello, world!
4.2 使用join()
方法
join()
方法可以将一个可迭代对象(如列表)中的元素连接成一个字符串,元素之间用指定的分隔符分隔。
示例:
words = ["Hello", "world"] sentence = " ".join(words) + "!" print(sentence) # 输出: Hello world!
相关问题与解答
问题1: 如何将字符串中的所有字母转换为大写?
解答: 可以使用upper()
方法将所有字母转换为大写。
示例:
text = "hello, world!" upper_text = text.upper() print(upper_text) # 输出: HELLO, WORLD!
问题2: 如何检查字符串是否以特定的子字符串开头或结尾?
解答: 可以使用startswith()
和endswith()
方法分别检查字符串是否以特定的子字符串开头或结尾。
示例:
text = "Hello, world!" print(text.startswith("Hello")) # 输出: True print(text.endswith("world!")) # 输出: True
以上内容就是解答有关“串处理语言翻译”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。