csharp,string connectionString = "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;";,
``MySQL连接字符串是用于建立与MySQL数据库连接的一组参数,这些参数通常包括主机名或IP地址、端口号、用户名、密码以及要连接的数据库名称等,通过这些参数,应用程序可以向MySQL服务器请求连接,并在成功连接后执行各种数据库操作。
一、MySQL连接字符串的构成

1、协议/驱动程序:指定使用的数据库驱动程序,例如jdbc:mysql://
表示使用JDBC驱动程序。
2、服务器地址:指定数据库服务器的地址,可以是IP地址或者域名。
3、端口号:指定数据库服务器的监听端口号,默认为3306。
4、数据库名称:指定要连接的数据库的名称。
5、用户名和密码:连接数据库所需要使用的用户名和密码。
二、示例代码
Python中的MySQL连接字符串
import mysql.connector 定义连接字符串 config = { 'user': 'root', 'password': 'password', 'host': 'localhost', 'database': 'mydatabase', 'port': '3306' } 建立数据库连接 try: connection = mysql.connector.connect(**config) # 连接成功 print("Connected to the database!") except mysql.connector.Error as e: # 连接失败 print("Failed to connect to the database!") print(e) finally: # 关闭数据库连接 if connection.is_connected(): connection.close() print("Connection closed.")
Java中的MySQL连接字符串
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class MySQLConnectionExample { public static void main(String[] args) { // 定义连接字符串 String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; // 建立数据库连接 try (Connection connection = DriverManager.getConnection(url, username, password)) { // 连接成功 System.out.println("Connected to the database!"); } catch (SQLException e) { // 连接失败 System.out.println("Failed to connect to the database!"); e.printStackTrace(); } } }
三、常见问题与解答
1、Q1:如何在MySQL连接字符串中指定字符集?
A1:在MySQL连接字符串中,可以通过添加charset
参数来指定字符集,在Python中使用charset='utf8mb4'
,在Java中使用useUnicode=true&characterEncoding=UTF-8
,这样可以确保在数据库操作中使用正确的字符编码,避免乱码问题。
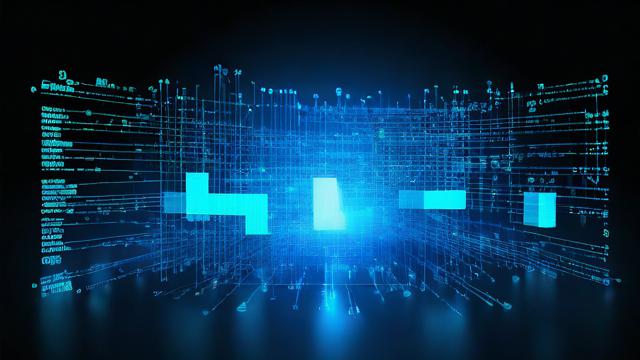
2、Q2:如何在MySQL连接字符串中启用SSL加密?
A2:在MySQL连接字符串中,可以通过添加ssl
参数并设置其值为true
来启用SSL加密,在Python中使用ssl=True
,在Java中使用ssl=true
,还可以通过指定SSL证书文件的位置来进一步配置SSL连接,这样可以提高数据库连接的安全性,防止数据在传输过程中被窃取或篡改。
小伙伴们,上文介绍了“c连接mysql连接字符串”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。