在ASP.X(通常指的是ASP.NET)中,遍历操作是一个常见的需求,无论是遍历集合、数组还是其他数据结构,以下是关于如何在ASP.NET中实现遍历的详细说明,包括使用循环、LINQ以及递归方法。
使用循环遍历
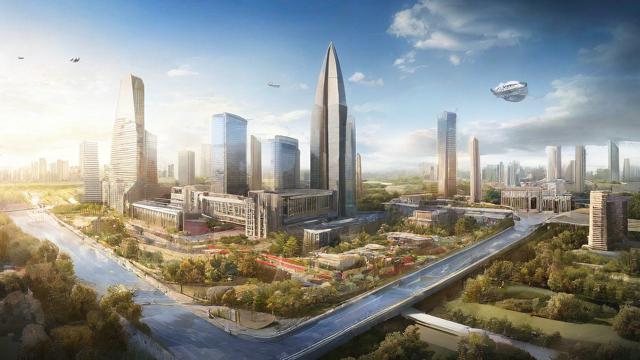
1.1. 遍历数组
在ASP.NET中,可以使用for
循环或foreach
循环来遍历数组。
using System; class Program { static void Main() { int[] numbers = { 1, 2, 3, 4, 5 }; // 使用 for 循环 for (int i = 0; i < numbers.Length; i++) { Console.WriteLine(numbers[i]); } // 使用 foreach 循环 foreach (int number in numbers) { Console.WriteLine(number); } } }
1.2. 遍历列表
对于列表,同样可以使用for
循环和foreach
循环进行遍历。
using System; using System.Collections.Generic; class Program { static void Main() { List<string> fruits = new List<string> { "Apple", "Banana", "Cherry" }; // 使用 for 循环 for (int i = 0; i < fruits.Count; i++) { Console.WriteLine(fruits[i]); } // 使用 foreach 循环 foreach (string fruit in fruits) { Console.WriteLine(fruit); } } }
使用LINQ遍历
LINQ(Language Integrated Query)提供了一种简洁且功能强大的方式来遍历和操作集合。
using System; using System.Collections.Generic; using System.Linq; class Program { static void Main() { List<int> numbers = new List<int> { 1, 2, 3, 4, 5 }; // 使用 LINQ 的 ForEach 方法 numbers.ForEach(number => Console.WriteLine(number)); } }
使用递归遍历
递归是一种函数调用自身的编程技术,常用于遍历树形结构或复杂数据结构。
using System; using System.Collections.Generic; class Program { static void Main() { List<int> numbers = new List<int> { 1, 2, 3, 4, 5 }; RecursiveTraverse(numbers, 0); } static void RecursiveTraverse(List<int> list, int index) { if (index >= list.Count) return; Console.WriteLine(list[index]); RecursiveTraverse(list, index + 1); } }
相关问题与解答
问题1:如何在ASP.NET中高效地遍历大型数据集?
解答1: 在ASP.NET中,遍历大型数据集时,效率非常重要,以下是几种提高遍历效率的方法:
使用IEnumerable和yield关键字: 这种方法可以延迟执行,只在需要时才生成元素,节省内存。
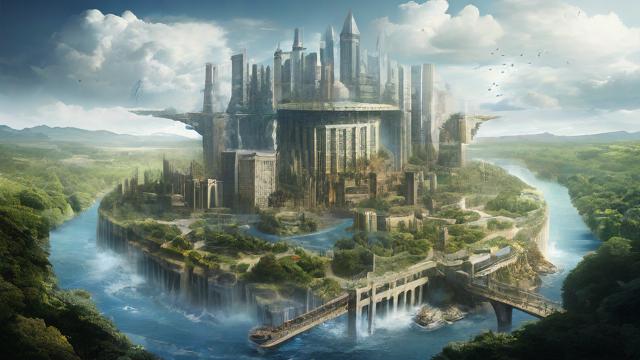
分页处理: 如果数据集非常大,可以考虑将其分页处理,每次只处理一部分数据。
并行处理: 使用Parallel.ForEach
等并行处理技术,可以充分利用多核CPU的优势,加快遍历速度。
优化查询: 确保在遍历之前已经对数据集进行了必要的过滤和排序,以减少遍历时的计算量。
问题2:在ASP.NET中,如何遍历一个嵌套的字典结构?
解答2: 遍历嵌套字典结构可以通过递归方法来实现,以下是一个示例:
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, object> nestedDict = new Dictionary<string, object>() { { "key1", 1 }, { "key2", new Dictionary<string, object>() { { "nestedKey1", 2 }, { "nestedKey2", 3 } } }, { "key3", new Dictionary<string, object>() { { "deepNestedKey", 4 } } } }; TraverseNestedDict(nestedDict); } static void TraverseNestedDict(object obj, string prefix = "") { if (obj is Dictionary<string, object> dict) { foreach (var kvp in dict) { TraverseNestedDict(kvp.Value, $"{prefix}{kvp.Key}."); } } else if (obj is int value) { Console.WriteLine($"{prefix}: {value}"); } } }
在这个示例中,我们定义了一个嵌套的字典结构,并使用递归方法TraverseNestedDict
来遍历整个结构,这个方法会检查每个元素的类型,如果是字典则继续递归,如果是整数则打印出来。
以上就是关于“aspx遍历”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!