解析JSON数据在C语言中是一个常见的任务,特别是在处理网络通信和配置文件时,本文将详细介绍如何在C语言中解析JSON数据,包括使用第三方库、手动解析以及相关示例代码。
JSON简介
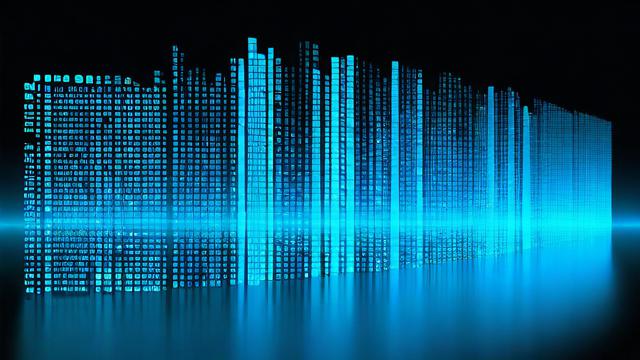
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人类阅读和编写,同时也便于机器解析和生成,JSON采用键值对的形式表示数据,其中键是字符串,值可以是字符串、数字、布尔值、数组或对象。
使用第三方库解析JSON
在C语言中,可以使用多个第三方库来解析JSON数据,如cJSON、json-c和Jansson,这些库提供了丰富的API,简化了JSON数据的解析和生成过程。
cJSON库
cJSON是一个轻量级的JSON解析库,适用于C语言,它支持解析JSON对象、数组、字符串、数字和布尔值,以下是一个简单的示例:
#include <stdio.h> #include <stdlib.h> #include "cJSON.h" int main() { const char *json_string = "{\"name\":\"John\", \"age\":30, \"is_student\":false}"; // 解析JSON字符串 cJSON *json = cJSON_Parse(json_string); if (json == NULL) { const char *error_ptr = cJSON_GetErrorPtr(); if (error_ptr != NULL) { fprintf(stderr, "Error before: %s ", error_ptr); } return -1; } // 获取并打印JSON数据 const cJSON *name = cJSON_GetObjectItemCaseSensitive(json, "name"); if (cJSON_IsString(name) && (name->valuestring != NULL)) { printf("Name: %s ", name->valuestring); } const cJSON *age = cJSON_GetObjectItemCaseSensitive(json, "age"); if (cJSON_IsNumber(age)) { printf("Age: %d ", age->valueint); } const cJSON *is_student = cJSON_GetObjectItemCaseSensitive(json, "is_student"); if (cJSON_IsBool(is_student)) { printf("Is student: %s ", cJSON_IsTrue(is_student) ? "true" : "false"); } // 释放内存 cJSON_Delete(json); return 0; }
json-c库
json-c是一个功能更全面的JSON解析库,支持更多的JSON特性,以下是一个使用json-c的示例:
#include <stdio.h> #include <json-c/json.h> int main() { const char *json_string = "{\"name\":\"John\", \"age\":30, \"is_student\":false}"; // 解析JSON字符串 struct json_object *json = json_tokener_parse(json_string); if (json == NULL) { fprintf(stderr, "Error parsing JSON "); return -1; } // 获取并打印JSON数据 struct json_object *name = json_object_get(json, "name"); if (!json_object_is_type(name, json_type_null)) { printf("Name: %s ", json_object_get_string(name)); } struct json_object *age = json_object_get(json, "age"); if (!json_object_is_type(age, json_type_null)) { printf("Age: %d ", json_object_get_int(age)); } struct json_object *is_student = json_object_get(json, "is_student"); if (!json_object_is_type(is_student, json_type_null)) { printf("Is student: %s ", json_object_get_boolean(is_student) ? "true" : "false"); } // 释放内存 json_decref(json); return 0; }
手动解析JSON
虽然使用第三方库可以简化JSON解析的过程,但在某些情况下,可能需要手动解析JSON数据,以下是一个手动解析简单JSON对象的示例:
#include <stdio.h> #include <stdlib.h> #include <string.h> typedef struct { char name[50]; int age; int is_student; } Person; void parse_json(const char *json, Person *person) { sscanf(json, "{\"name\":\"%[^\"]\", \"age\":%d, \"is_student\":%d}", person->name, &person->age, &person->is_student); } int main() { const char *json_string = "{\"name\":\"John\", \"age\":30, \"is_student\":false}"; Person person; parse_json(json_string, &person); printf("Name: %s ", person.name); printf("Age: %d ", person.age); printf("Is student: %s ", person.is_student ? "true" : "false"); return 0; }
相关问题与解答
问题1:如何在C语言中使用cJSON库生成JSON数据?
解答: 使用cJSON库生成JSON数据非常简单,以下是一个示例代码,演示如何创建一个简单的JSON对象并将其转换为字符串:
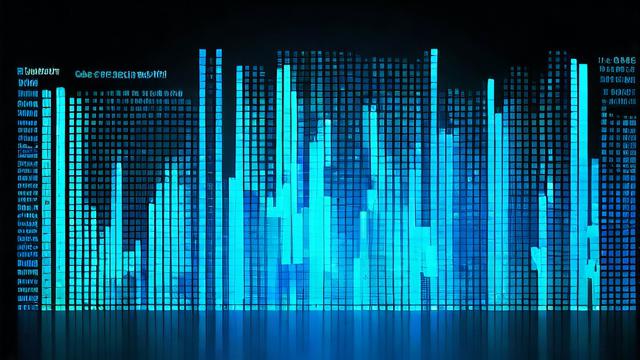
#include <stdio.h> #include <stdlib.h> #include "cJSON.h" int main() { // 创建一个JSON对象 cJSON *json = cJSON_CreateObject(); if (json == NULL) { fprintf(stderr, "Error creating JSON object "); return -1; } // 添加键值对到JSON对象 cJSON_AddStringToObject(json, "name", "John"); cJSON_AddNumberToObject(json, "age", 30); cJSON_AddBoolToObject(json, "is_student", cJSON_False); // 将JSON对象转换为字符串 char *json_string = cJSON_Print(json); if (json_string == NULL) { fprintf(stderr, "Error printing JSON string "); cJSON_Delete(json); return -1; } // 打印JSON字符串 printf("%s ", json_string); // 释放内存 free(json_string); cJSON_Delete(json); return 0; }
问题2:如何处理复杂的嵌套JSON结构?
解答: 处理复杂的嵌套JSON结构需要递归地解析各个层级的数据,以下是一个示例代码,演示如何解析一个包含嵌套对象的JSON字符串:
#include <stdio.h> #include <stdlib.h> #include "cJSON.h" typedef struct { char address[100]; int postal_code; } Address; typedef struct { char name[50]; int age; Address address; } Person; void parse_address(const cJSON *address_json, Address *address) { cJSON *street = cJSON_GetObjectItemCaseSensitive(address_json, "street"); if (cJSON_IsString(street) && (street->valuestring != NULL)) { strncpy(address->address, street->valuestring, sizeof(address->address) 1); } cJSON *postal_code = cJSON_GetObjectItemCaseSensitive(address_json, "postal_code"); if (cJSON_IsNumber(postal_code)) { address->postal_code = postal_code->valueint; } } void parse_person(const cJSON *person_json, Person *person) { cJSON *name = cJSON_GetObjectItemCaseSensitive(person_json, "name"); if (cJSON_IsString(name) && (name->valuestring != NULL)) { strncpy(person->name, name->valuestring, sizeof(person->name) 1); } cJSON *age = cJSON_GetObjectItemCaseSensitive(person_json, "age"); if (cJSON_IsNumber(age)) { person->age = age->valueint; } cJSON *address_json = cJSON_GetObjectItemCaseSensitive(person_json, "address"); if (cJSON_IsObject(address_json)) { parse_address(address_json, &person->address); } } int main() { const char *json_string = "{\"name\":\"John\", \"age\":30, \"address\":{\"street\":\"123 Main St\", \"postal_code\":12345}}"; cJSON *json = cJSON_Parse(json_string); if (json == NULL) { const char *error_ptr = cJSON_GetErrorPtr(); if (error_ptr != NULL) { fprintf(stderr, "Error before: %s ", error_ptr); } return -1; } Person person; parse_person(json, &person); printf("Name: %s ", person.name); printf("Age: %d ", person.age); printf("Address: %s ", person.address.address); printf("Postal Code: %d ", person.address.postal_code); cJSON_Delete(json); return 0; }
各位小伙伴们,我刚刚为大家分享了有关“c语音解析json”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!